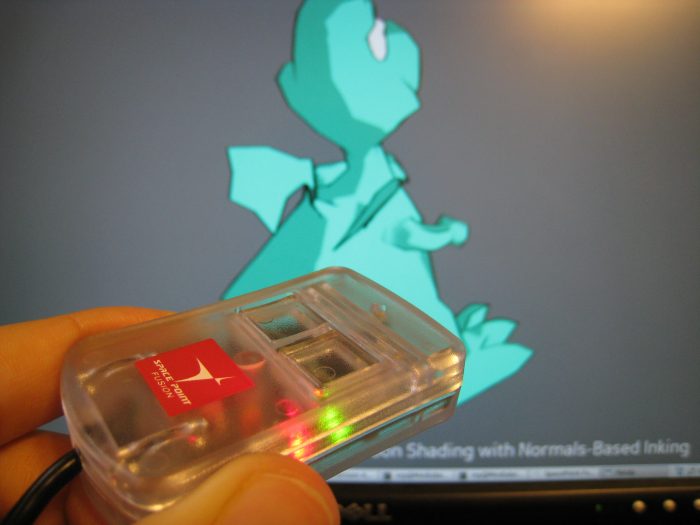
My college roommate Donnie mentioned the PNI SpacePoint Fusion in comments of the HMC5843 post, and it seemed too good to be true. A 9 DOF controller (3 axes each of magnetometer, accelerometer, and gyro) with a Kalman filter to calculate a smooth quaternion that interfaces as a USB HID device, all for under $100. I’d be surprised if PNI is making any profit on it. I sound more like a shill than I’m normally comfortable with, but I’m truly impressed with this gadget. I have some plans for it involving a Microvision SHOWWX that I’m quite excited about; I’ll write more on that when it’s available in a couple of months.
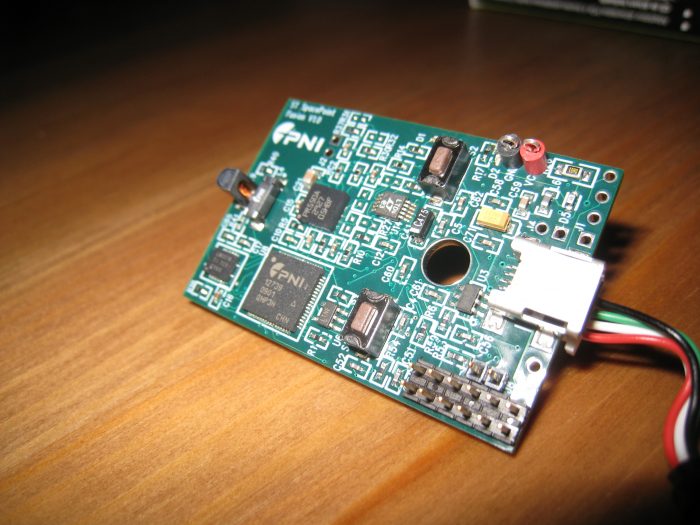
PNI provides some Windows only sample apps that show off how weirdly stable and precise the SpacePoint Fusion is. Luckily, since it’s a normal USB HID device (redundant, I know), and PNI provides application notes, it’s easy to use on any platform. I wrote a Python module that uses libhid via python-hid to make it easy to prototype with in Linux. The usage is pretty simple, as shown below. Note that when plugging the device in, you need to keep it still for a few seconds while the gyros are calibrated. After that, the quaternion, accelerometer, and button data can be updated 62.5 times a second.
>>> import spacepoint
>>> fusion = spacepoint.SpacePoint()
>>> print repr(fusion.quat)
(0.987518310546875, -0.04425048828125, -0.04119873046875, 0.145294189453125)
>>> print repr(fusion.accel)
(-0.054016113354999999, 0.018859863306999999, -0.89648437622400001)
>>> print repr(fusion.buttons)
(0, 0)
>>> fusion.update()
>>> print repr(fusion)
accel: (-0.054016113354999999, 0.018859863306999999, -0.89648437622400001)
quat: (0.97186279296875, -0.233428955078125, -0.030548095703125, 0.005401611328125)
buttons: (0, 0) |
>>> import spacepoint
>>> fusion = spacepoint.SpacePoint()
>>> print repr(fusion.quat)
(0.987518310546875, -0.04425048828125, -0.04119873046875, 0.145294189453125)
>>> print repr(fusion.accel)
(-0.054016113354999999, 0.018859863306999999, -0.89648437622400001)
>>> print repr(fusion.buttons)
(0, 0)
>>> fusion.update()
>>> print repr(fusion)
accel: (-0.054016113354999999, 0.018859863306999999, -0.89648437622400001)
quat: (0.97186279296875, -0.233428955078125, -0.030548095703125, 0.005401611328125)
buttons: (0, 0)
Update on February 7, 2010: I emailed PNI about a bug in the firmware, and got the following response:
Thank you for submitting the SpacePoint bug regarding libusb, Python, and Linux. You’re right! There is a bug in the SpacePoint FW that prevented opening interface 1 without opening interface 0 when using libusb under Linux. While your work around was effective in allowing the device to operate normally, one should be able to open interface 1 directly without the work around. We were able to use your Python source code to quickly diagnose and repair the bug. Please see the attached for the modified Python script. Please feel free to post this paragraph, the modified code, and all bragging rights on your blog (https://eclecti.cc/).
Units being shipped now have the fix. I modified the Python module to handle units both with and without the firmware fix and bumped the version to 0.2.
Download:
SpacePoint Python Module or
Standalone spacepoint.py
Setting udev rules to get the permissions right
In most cases, the module will just work properly. However, if you get the following error, you probably don’t have the right permissions to access the usb device.
>>> import spacepoint
>>> fusion = spacepoint.SpacePoint()
hid_force_open failed with return code 12. |
>>> import spacepoint
>>> fusion = spacepoint.SpacePoint()
hid_force_open failed with return code 12.
On most modern Linux distros, you can fix this by setting a udev rule for the device. In Ubuntu Karmic Koala, saving the following as /etc/udev/rules.d/45-spacepoint.rules
, running sudo service udev restart
, and then unplugging and replugging in the device should fix it:
# PNI SpacePoint Fusion
SYSFS{idVendor}=="20ff", SYSFS{idProduct}=="0100", MODE="0664", GROUP="admin" |
# PNI SpacePoint Fusion
SYSFS{idVendor}=="20ff", SYSFS{idProduct}=="0100", MODE="0664", GROUP="admin"